Getting Started with Docker compose: A Beginner’s Guide
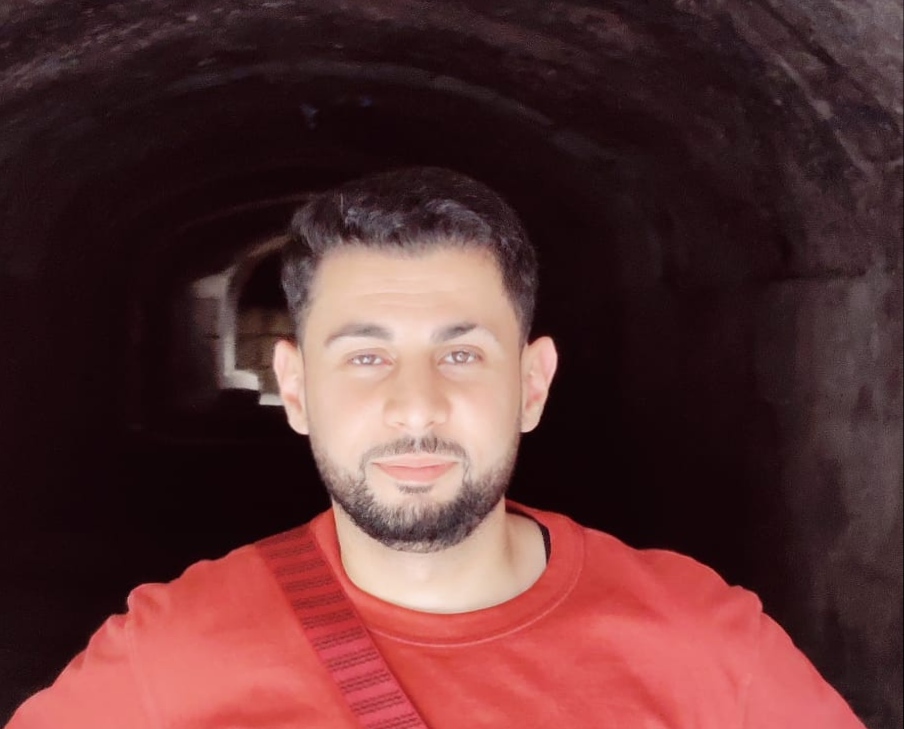
MontaF - Sept. 12, 2024
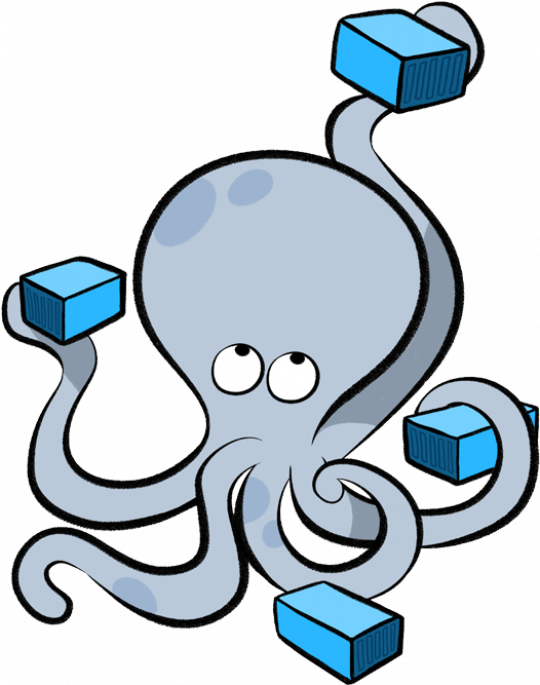
Docker Compose is a tool for defining and running multi-container Docker applications. With Compose, you use a YAML file to configure your application’s services, networks, and volumes, and then run it with a single command. Here's how to get started with Docker Compose:
1. Install Docker and Docker Compose
Before starting, you need Docker and Docker Compose installed on your machine.
a.Docker: Follow the instructions to install Docker from Docker's official site.
b.Docker Compose: Docker Desktop comes with Docker Compose pre-installed, but you can check your installation by running:
docker-compose --version
2. Create a Project Directory
First, create a directory for your project:
mkdir my-docker-app
cd my-docker-app
3. Define Your Application’s Services
In your project directory, create a file named docker-compose.yml
which will define the services in your app.
For example, if you’re building a simple web app with a Python backend and Redis as a database, your docker-compose.yml
might look like this:
version: '3'
services:
web:
image: python:3.9
volumes:
- .:/app
working_dir: /app
command: python app.py
ports:
- "5000:5000"
depends_on:
- redis
redis:
image: "redis:alpine"
- version: Defines the version of Docker Compose.
- services: Defines the individual containers for your application. In this example, we have a
web
service and aredis
service. - web service: Runs a Python application and maps the container’s port
5000
to the host machine’s port5000
. - redis service: Runs a Redis database using the
redis:alpine
image.
4. Create Your Application Code
Inside your project folder, create a simple Python application (app.py
):
import time
import redis
from flask import Flask
app = Flask(__name__)
cache = redis.Redis(host='redis', port=6379)
def get_hit_count():
retries = 5
while True:
try:
return cache.incr('hits')
except redis.exceptions.ConnectionError as exc:
if retries == 0:
raise exc
retries -= 1
time.sleep(0.5)
@app.route('/')
def hello():
count = get_hit_count()
return f"Hello World! I have been seen {count} times.\n"
if __name__ == "__main__":
app.run(host="0.0.0.0", port=5000)
This app connects to the Redis service and counts the number of times the homepage has been accessed.
5. Build and Run the Application
To start your application, navigate to your project directory and run:
docker-compose up
This command:
- Downloads the necessary images (Python and Redis in this case).
- Builds the web service (if required).
- Starts both services (web and redis).
You should see logs from both services. Once the services are running, open your web browser and go to http://localhost:5000
. You should see the “Hello World” message along with the count of visits.
6. Access Running Containers
You can access the logs of any running container using:
docker-compose logs
If you want to run a command inside a running container (e.g., to access the shell of the web
service), you can do:
docker-compose exec web bash
7. Stop the Application
To stop the running containers, simply run:
docker-compose down
This command will stop and remove all the containers.
Advanced Topics:
Scaling Services: You can scale any service by running:
docker-compose up --scale web=3
This command will scale the web
service to 3 instances.
Using Volumes: In the docker-compose.yml
file, volumes allow you to persist data or bind a host directory to a container directory.
Environment Variables: You can pass environment variables to services by using the environment
section in docker-compose.yml
or by using .env
files.
Conclusion
Docker Compose simplifies the process of managing multi-container applications. It's especially useful when working on projects that require multiple services like a web server, database, and caching system. You can now deploy your app, scale it, and manage services easily!
Let me know if you'd like help with a more complex example or any specific configuration!