How to Test Your Python Application with pytest!
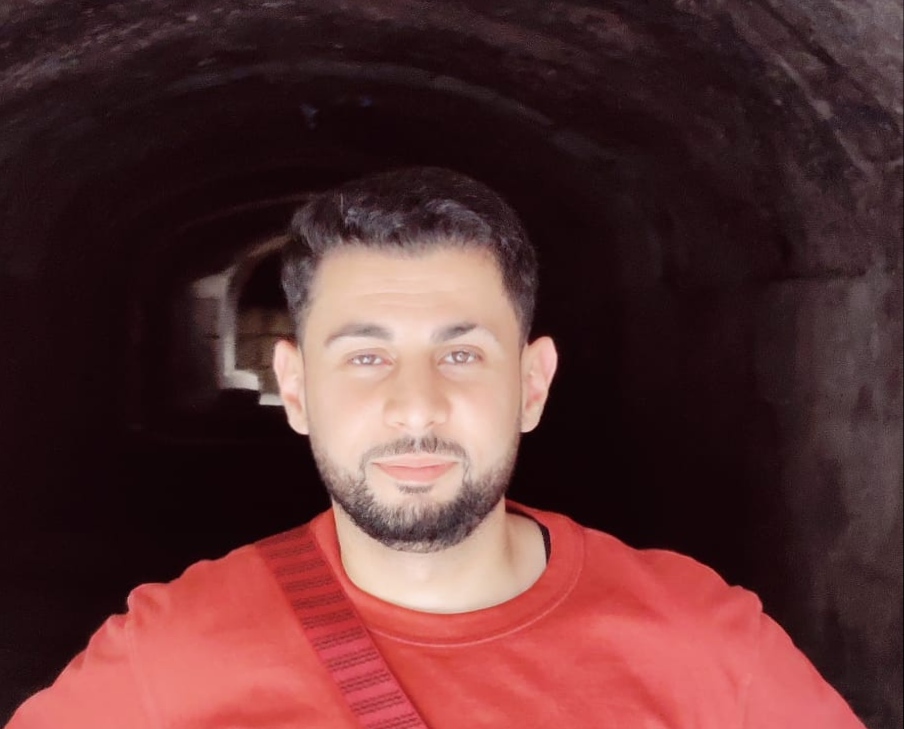
MontaF - Sept. 30, 2024
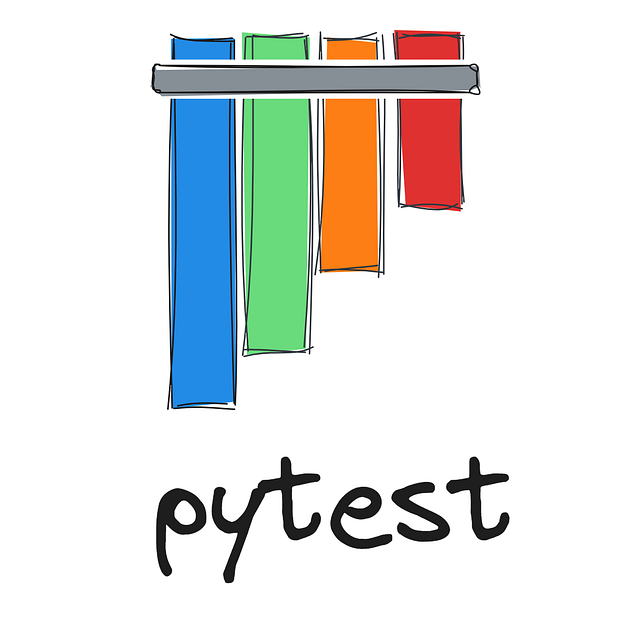
Let’s be real: testing your code isn’t the glamorous part of development, but it is essential. And trust me—if you’ve ever had to troubleshoot a bug that somehow only shows up at 3 AM, pytest is here to make sure that never happens again.
In this Blog, we’re diving deep into pytest, the Python testing framework that’s as flexible as it is powerful. We’ll explore:
- Fixtures
- conftest.py
- Factories
- Patches
- Parametrize
- Mocks
- Generators
- Assert Statements
- Stubs
- Test Discovery
- Pytest Plugins
- Code Coverage
- Testing Exceptions
- Markers
- Test Ordering
- Continuous Integration
We’ll keep things practical and straightforward.
1. Fixtures: The Backbone of pytest
Fixtures are like the well-organized toolbox every developer wishes they had. They allow you to set up the environment before each test runs, ensuring your tests are always clean and repeatable.
Example:
import pytest @pytest.fixture def database(): return {"user": "admin", "password": "secret"} def test_database_connection(database): assert database["user"] == "admin"
Fixtures make it easy to maintain reusable, isolated test data. If you’re running a test suite with multiple components, using fixtures is a must to keep things organized and prevent data contamination.
2. conftest.py: Sharing Fixtures Across Your Tests
Tired of copying the same setup code into every test file? That’s where conftest.py
steps in. It’s like the Robin to your pytest’s Batman, enabling you to define reusable fixtures across your entire project.
Create a conftest.py
file and define shared fixtures:
# conftest.py import pytest @pytest.fixture def user(): return {"username": "test_user"}
Now any test can access the user
fixture without needing to import it, making your life a lot easier.
3. Factories: Turbocharging Data Generation
If fixtures are your toolkit, factories are your 3D printer. They allow you to generate test data dynamically, on demand. This is incredibly useful when you’re testing code that deals with databases or APIs.
Example:
class UserFactory: def create_user(username): return {"username": username, "active": True} def test_user(): user = UserFactory.create_user("codemaster") assert user["username"] == "codemaster"
With factories, you can produce a variety of test data with ease.
4. Patching: Altering Reality for Your Tests
Sometimes, your test code interacts with external APIs or services that you don’t want to hit during tests. Enter patching—the way to mock out real functions or classes.
Let’s say you want to test code that sends an email:
from unittest.mock import patch @patch('email_service.send_email') def test_email(mock_send): mock_send.return_value = True assert email_service.send_email() is True
By patching, you bypass the real email sending process while still verifying that your code behaves correctly.
5. Parametrize: Running a Single Test with Multiple Inputs
The @pytest.mark.parametrize
decorator is like a buffet for your tests: why settle for just one set of inputs when you can feast on many?
Here’s how it works:
@pytest.mark.parametrize("a, b, expected", [(1, 2, 3), (10, 20, 30), (-5, 5, 0)]) def test_add(a, b, expected): assert a + b == expected
This one test will now run three times, with different inputs each time. It’s a great way to make your tests leaner and meaner.
6. Mocks: Faking It Like a Pro
Mocks allow you to replace parts of your system under test with dummy implementations, giving you full control over how those parts behave.
Example:
from unittest.mock import Mock def test_mock(): mock = Mock() mock.some_method.return_value = "Mocked!" assert mock.some_method() == "Mocked!"
Mocks are perfect for isolating the unit of code you’re testing from external dependencies.
7. Generators: Keep It Light with Lazy Data
Generators in pytest are like a low-carb diet for your tests: they give you what you need without the heavy baggage of holding everything in memory. This is particularly useful for testing large datasets.
Example:
def data_generator(): for i in range(5): yield i def test_generator(): gen = data_generator() assert next(gen) == 0 assert next(gen) == 1
Generators help you write efficient tests, even when dealing with tons of data.
8. Assert Statements: Leveling Up Your Assertions
One of the best features of pytest is its ability to provide detailed error messages when an assertion fails. No more AssertionError
with no context!
Example:
def test_assertions(): a, b = 2, 3 assert a + b == 5, "Math is broken!"
When this test fails, pytest tells you exactly what went wrong, down to the values of a
and b
.
9. Stubs: Minimal, Lightweight, Perfect for Quick Fixes
Sometimes, you don't need the full complexity of mocking—just a lightweight stand-in for a function. That’s where stubs come in. They allow you to fake a specific function's behavior in your tests without the overhead of full mock objects.
Example:
def stub_function(): return "stubbed!" def test_stub(): result = stub_function() assert result == "stubbed!"
10. Test Discovery: Pytest Finds Your Tests, You Enjoy Coffee
Pytest makes discovering and running your tests a breeze. It automatically looks for files starting with test_
or ending with _test.py
and runs the functions inside them that start with test_
.
Pro Tip: You can customize test discovery with options like --maxfail
(stop after N failures) or -k
(run tests matching a keyword).
11. Pytest Plugins: Add Superpowers to Your Testing
Did you know pytest has plugins for everything? Running tests in parallel, capturing logs, generating reports—you name it. With plugins like pytest-xdist
, you can run tests on multiple CPUs, or use pytest-cov
for coverage reports.
Pro Tip: Check out pytest's plugin registry for endless possibilities!
12. Code Coverage: How Much Have You Tested?
How much of your code is actually covered by your tests? With pytest-cov
, you can generate coverage reports and make sure you’re testing all the important stuff (and not leaving gaping holes in your code’s armor).
Example:
pytest --cov=your_project
This will show you what percentage of your code is covered. Aim high—because 100% coverage means your code is invincible (in theory).
13. Testing Exceptions: Let’s Break Some Stuff
Testing exceptions is as important as testing normal flows. With pytest, you can check if your code throws the expected exceptions, ensuring it behaves correctly when things go sideways.
Example:
import pytest def test_exception(): with pytest.raises(ZeroDivisionError): 1 / 0
14. Markers: Flagging Tests for Special Treatment
You can flag tests with markers to tell pytest how to treat them. Whether you want to skip certain tests, mark some as slow, or even categorize tests into custom groups, markers make it easy.
Example:
@pytest.mark.skip(reason="Work in progress") def test_incomplete(): assert 1 + 1 == 2
15. Test Ordering: Control the Chaos
Usually, pytest runs tests in a random order, but sometimes you need control over this. Maybe one test depends on another, or you want to run a specific test first.
Pro Tip: Use pytest-ordering
to define the order in which tests should be run.
pip install pytest-ordering
16. Continuous Integration with Pytest
Once you’ve written your tests, why not let machines run them for you every time you push to GitHub or GitLab? Integrate pytest with a CI/CD tool like GitHub Actions or Jenkins to run tests automatically after every commit. No human intervention required!
Conclusion: Pytest—Your Ultimate Weapon in the War Against Bugs
By now, you’re armed with knowledge on everything from fixtures and factories to patches, mocks, and generators. Add to that test discovery, parametrize, code coverage, and pytest plugins, and you have an unstoppable testing machine. Whether you’re squashing bugs or preventing new ones, pytest has your back.
So, what are you waiting for? Run your tests, catch those bugs, and earn your title as a Testing Ninja!