How to Ace a Coding Interview and Land Your Dream Job
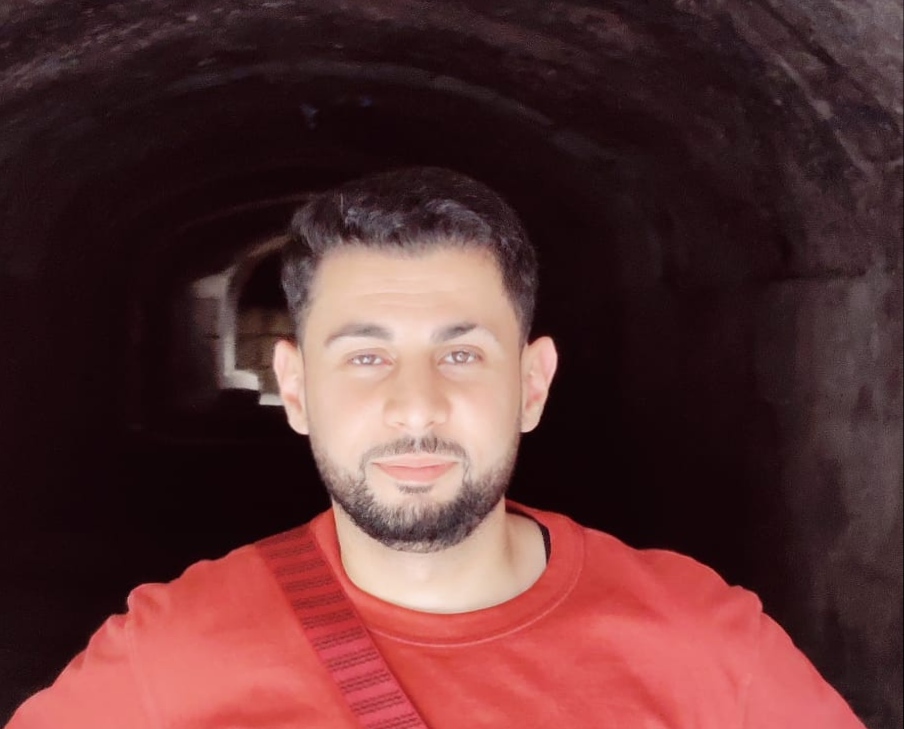
MontaF - Oct. 3, 2024
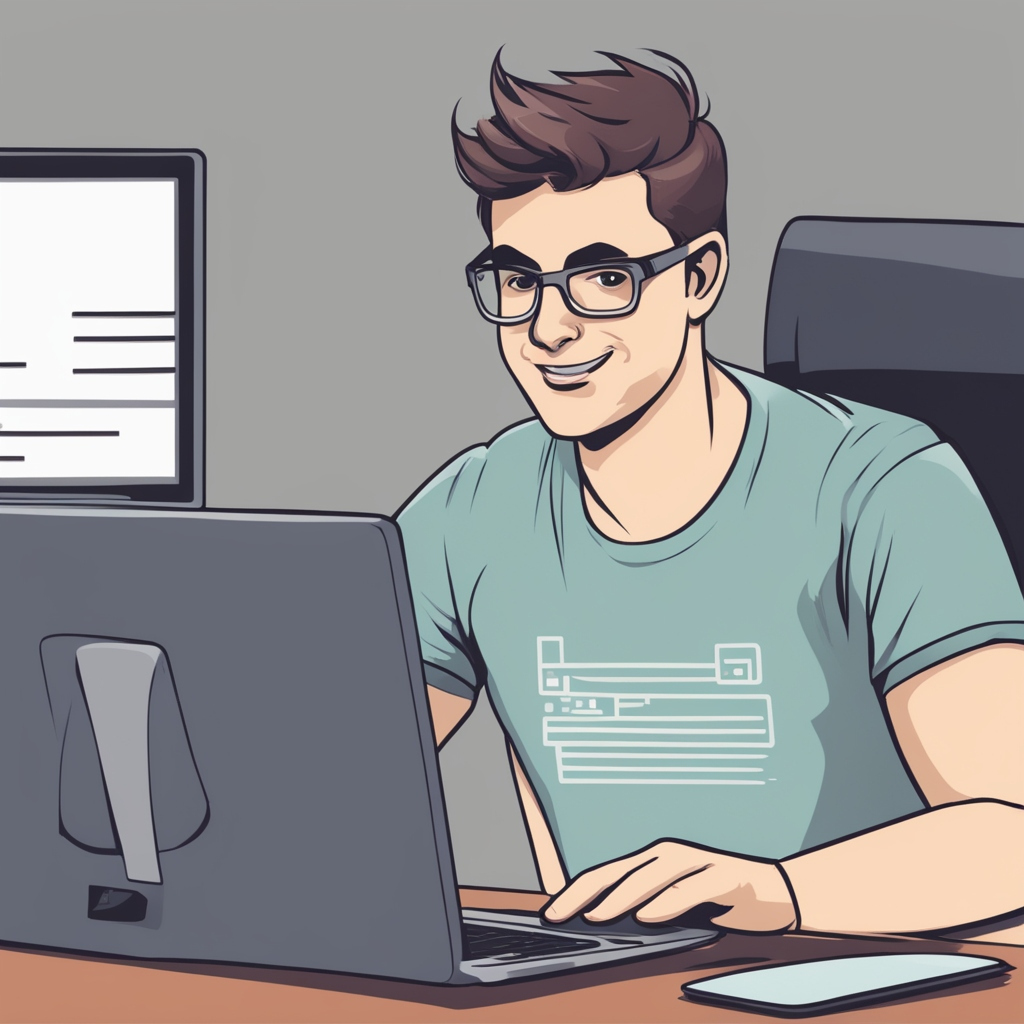
You’ve polished your résumé, bought a new shirt, and practiced saying "synergy" without giggling. Great! But there's one more thing standing between you and that dream job: the coding interview. Coding interviews can be nerve-wracking, but don’t worry—you’re about to learn how to tackle them like a pro. With a little humor and some Python magic, you'll be well on your way to impressing even the toughest interviewers.
Let’s dive into the secrets of acing that coding interview!
1. Know the Basics—Like Really Know Them 🧠
You might be tempted to dive into algorithms and data structures, but first, make sure you’re solid on the basics. If you walk into an interview and freeze up when asked to reverse a string, it won’t matter if you can implement Dijkstra’s algorithm in your sleep.
Example:
# Reverse a string in Python def reverse_string(s): return s[::-1] # Yes, it's that easy in Python. print(reverse_string("hello")) # Output: 'olleh'
Knowing simple things like slicing in Python can save you time and impress interviewers with your fluency in the language. (Plus, it's fun to look like a wizard when you write one-liners.)
Pro tip: Even if it's easy, explain your thought process clearly. You want to come off as someone who not only knows their stuff but can communicate it effectively.
2. Understand Data Structures and Algorithms 🗂️
Data structures and algorithms are the bread and butter of coding interviews. You don’t need to memorize every obscure algorithm out there, but you should definitely know the essentials: arrays, linked lists, hashmaps, trees, graphs, and the algorithms associated with them.
Here’s a quick reminder: Big-O Notation is not some secret spy language—it’s just a way of measuring the efficiency of your code. (Imagine Big-O is your friend who helps you avoid writing code that takes 3 hours to run.)
Example: Sorting a list of numbers.
# QuickSort in Python def quicksort(arr): if len(arr) <= 1: return arr pivot = arr[len(arr) // 2] left = [x for x in arr if x < pivot] middle = [x for x in arr if x == pivot] right = [x for x in arr if x > pivot] return quicksort(left) + middle + quicksort(right) print(quicksort([3,6,8,10,1,2,1])) # Output: [1, 1, 2, 3, 6, 8, 10]
If the interviewer asks, "What’s the time complexity of QuickSort?" confidently say, "O(n log n) on average, but O(n²) in the worst case." (And try to suppress the fear that they might ask you to explain the worst case.)
3. Master Problem-Solving Patterns 🧩
Many coding problems follow certain patterns. Once you recognize these, solving problems becomes much easier. Some common patterns include:
- Two-pointer technique: Great for problems involving arrays or linked lists.
- Sliding window: Useful for substring or subarray problems.
- Divide and conquer: Break a problem into smaller pieces (like in QuickSort).
Example: Two-pointer technique to check if an array is a palindrome.
# is_palindrome in Python def is_palindrome(arr): left, right = 0, len(arr) - 1 while left < right: if arr[left] != arr[right]: return False left += 1 right -= 1 return True print(is_palindrome([1, 2, 3, 2, 1])) # Output: True
Interviewer: "Can you optimize this further?" You: "Hmm... no, but I could run faster if you buy me coffee!" ☕ (Don’t actually say this, unless the interview is going really well.)
4. Talk Through Your Solution 🎙️
Even if you’re quietly freaking out on the inside, try to sound calm and confident on the outside. Explain your thought process as you work through the problem. This isn’t just about the solution—interviewers want to see how you think.
- Start by clarifying the problem. "So, you want me to find the second largest number in this list?"
- Discuss edge cases. "What if the list only contains one number? Should I return an error?"
- Explain your approach. "I’m going to loop through the list and keep track of the largest and second-largest numbers."
5. Don’t Panic If You Don’t Know the Answer 😱
We’ve all been there—staring at a coding problem and feeling your brain go completely blank. First, breathe. You don’t have to know everything. What’s important is how you approach the problem.
Try to break it down into smaller steps. If you really don’t know where to start, ask the interviewer for hints. They might nudge you in the right direction, and it’s better than sitting there in silence.
Example: Stuck on recursion? Try brute force first!
# Brute force solution to find the nth Fibonacci number def fibonacci(n): if n <= 1: return n return fibonacci(n-1) + fibonacci(n-2) print(fibonacci(6)) # Output: 8
Then you can say, "I know this is not the most efficient solution, but I’ll optimize it using dynamic programming or memoization."
6. Optimize, But Don’t Obsess 🛠️
Once you’ve solved the problem, it’s time to optimize. But don’t worry if you don’t immediately come up with the most efficient solution. Interviewers are often happy to see a brute-force solution first, as long as you acknowledge its inefficiency and discuss how to improve it.
Example: Memoized Fibonacci.
# Optimized Fibonacci using memoization def fibonacci_memo(n, memo={}): if n in memo: return memo[n] if n <= 1: return n memo[n] = fibonacci_memo(n-1, memo) + fibonacci_memo(n-2, memo) return memo[n] print(fibonacci_memo(50)) # Output: 12586269025
Now you’re in Big-O heaven with O(n) complexity instead of O(2^n). Your interviewer’s eyes will light up like you just solved world hunger.
7. Practice, Practice, Practice 🏋️♂️
The best way to get good at coding interviews is practice. Start with our quizzes and platforms like codeforces (we'll soon add our problems section to give you a training environment). Set aside time each day to solve one or two problems. Over time, you’ll develop a feel for common interview questions.
Oh, and don’t forget mock interviews! Whether it's with a friend, a mentor, or your rubber duck, practice explaining your solutions out loud.
8. Behavioral Questions Matter Too 🧐
It’s not all about algorithms and data structures. You’ll likely face behavioral questions like:
- "Tell me about a time you faced a challenge at work."
- "How do you handle tight deadlines?"
Be ready with examples that highlight your problem-solving skills, teamwork, and adaptability. Also, don’t be afraid to show a bit of personality. Companies want to hire people they’d enjoy working with, not just coding machines.
9. Dress for Success (and Comfort) 👗👔
Finally, let’s not forget one of the most important interview tips: dressing appropriately. But make sure you’re comfortable too.
There’s no need to rock a tuxedo for a Zoom interview (unless that’s your style). Wear something that makes you feel confident and ready to take on the world—or at least a tricky coding problem.
Conclusion: Ace That Interview! 🎉
Coding interviews can be intimidating, but with the right preparation and mindset, you can turn them into an opportunity to showcase your skills. Remember to stay calm, explain your thought process, and practice regularly. Whether you’re debugging a hairy algorithm or just trying to reverse a string, the key is to keep learning and improving.
And who knows? After acing your interview, you might just be the one asking the questions in the future. 😉
Good luck, coder—go land that dream job! 🚀
Enjoyed the Read? 👏
If this blog helped you or made you laugh (even a little), give it a clap! Your support encourages us to keep bringing you more tips, tricks, and insights to ace your coding journey. 🙌
Share it with your friends—whether they’re prepping for interviews or looking to level up their skills. Every share helps us grow and allows us to add more awesome features to our platform.
Thank you for being part of our community—let’s build something amazing together! 🚀