Top 10 Secrets to Writing Clean and Maintainable Code
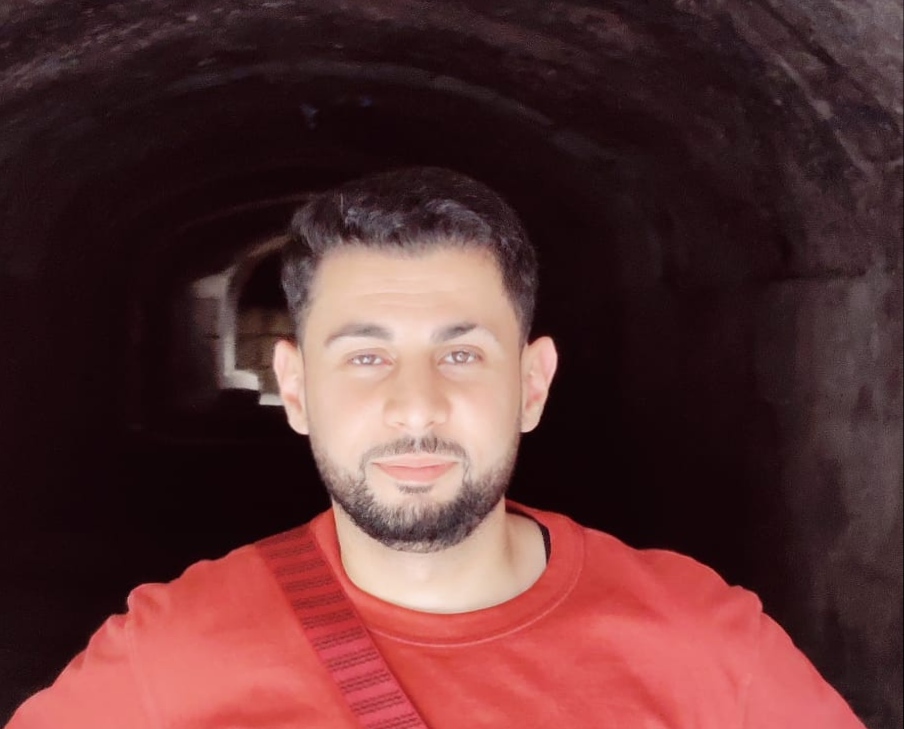
MontaF - Oct. 3, 2024
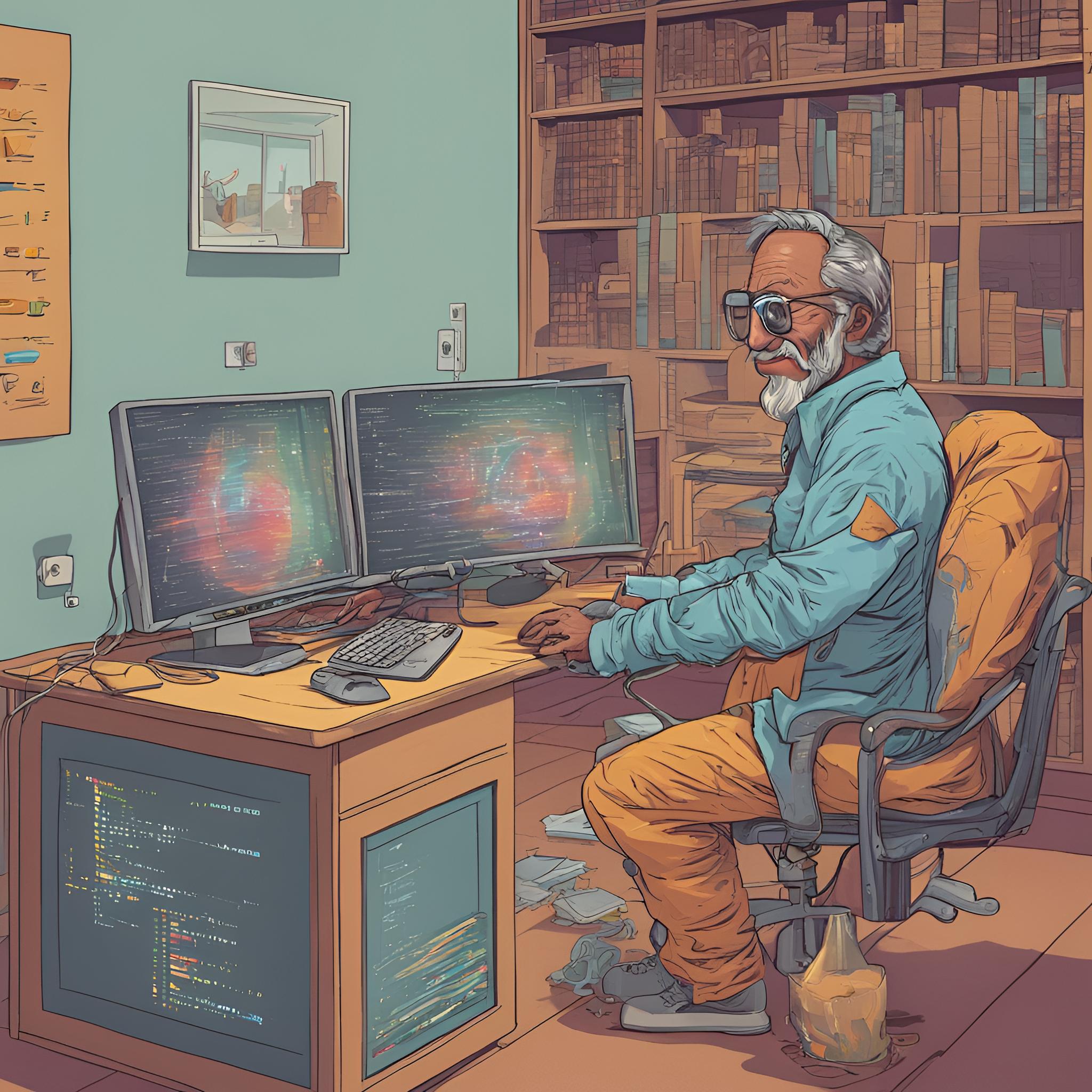
Ah, coding. It’s a beautiful art form... until you return to the masterpiece you wrote six months ago, and it looks like a spaghetti bowl threw up on your screen. Ever had that sinking feeling where you wonder, who wrote this mess only to realize—oh no, it was you?
Fear not, my fellow coder! In this blog, we’ll dive into the top 10 secrets to writing clean, maintainable code that won’t make your future self (or your teammates) cry. We'll keep it light, fun, and of course, filled with Python goodness!
So, grab your coffee (or tea) and let’s clean up that codebase, one function at a time.
1. Keep It DRY (Don’t Repeat Yourself) 🚿
First things first, repeat after me: I will not repeat myself. You’ll often encounter problems that seem so similar, you end up copying and pasting code snippets across your project. But beware! This leads to chaos when you need to update something.
Instead, encapsulate repeated logic into reusable functions or modules. This way, you only have to change things in one place when something breaks (and trust me, it will).
Example:
# Bad: Same logic repeated multiple times def send_email_to_user1(): # sending email logic def send_email_to_user2(): # sending email logic # Good: Encapsulate logic in a reusable function def send_email(user): # sending email logic send_email(user1) send_email(user2)
Your code should be like a well-structured IKEA manual—no unnecessary duplicate steps. Unless, of course, you’re into losing screws.
2. Meaningful Variable Names 📛
You’re not writing a secret code for an underground hacker group. Variables like x
, y
, and stuff
aren’t going to cut it. Naming things is hard, but if you put some effort into making your variables descriptive, your code will become much more readable.
Example:
# Bad x = 30 y = 20 z = x * y # Good width = 30 height = 20 area = width * height
Descriptive variables save you (and others) from the horror of playing “guess what this does” every time you revisit the code. Plus, future you will appreciate not having to decipher tempData2FinalFinal.py
.
3. Write Small, Single-Purpose Functions 🧩
You know that massive, 200-line function you just wrote? Yeah... let’s break that up. Functions should do one thing and do it well. Not 12 things poorly.
If your function is creeping toward novel-length, it’s time to split it up into smaller, manageable pieces.
Example:
Before Refactor: One Large Function Doing Too Much
# Bad: A giant function that handles multiple tasks def process_order(order): # Calculate total price with tax tax_rate = 0.05 total = 0 for item in order["items"]: total += item["price"] * item["quantity"] total_with_tax = total + (total * tax_rate) # Apply discount if available if "discount" in order: total_with_tax -= order["discount"] # Charge payment payment_processed = False if order["payment_info"]["card_valid"]: payment_processed = True # Send confirmation email if payment_processed: email = order["user_email"] print(f"Sending confirmation email to {email}") return total_with_tax, payment_processed
After Refactor: Breaking It Down into Small, Single-Purpose Functions
# Good: Break the logic into smaller, focused functions # Function to calculate total with tax def calculate_total(order): tax_rate = 0.05 total = sum(item["price"] * item["quantity"] for item in order["items"]) return total + (total * tax_rate) # Function to apply discount def apply_discount(total, discount): return total - discount if discount else total # Function to process payment def process_payment(payment_info): return payment_info["card_valid"] # Function to send confirmation email def send_confirmation_email(email): print(f"Sending confirmation email to {email}") # Main function orchestrating the smaller functions def process_order(order): total_with_tax = calculate_total(order) if "discount" in order: total_with_tax = apply_discount(total_with_tax, order["discount"]) payment_processed = process_payment(order["payment_info"]) if payment_processed: send_confirmation_email(order["user_email"]) return total_with_tax, payment_processed
If your code were a sandwich, it would be a neat stack of ingredients—not an overstuffed monstrosity that spills out everywhere.
4. Comment, But Not Too Much 📝
Comments are your friend, but like all friendships, there’s a delicate balance. Too few comments and no one knows what’s going on. Too many comments, and it feels like reading the entire Lord of the Rings trilogy just to understand a simple function.
Use comments to clarify why you did something, not what the code is doing (that should be obvious if you’ve followed steps 1-3).
Example:
# Bad: Over-commenting i = 0 # initializing variable i to 0 for i in range(0, 10): # looping from 0 to 10 print(i) # printing i # Good: Commenting the why # We need to iterate through all user IDs for processing for user_id in range(0, len(users)): process_user(user_id)
Comments should be like seasoning: enough to add flavor, but not so much that it overpowers the whole dish.
5. Avoid Magic Numbers 🔮
42. It’s the answer to the ultimate question of life, the universe, and everything. But what does it mean in your code? Probably nothing—unless you’ve defined what that magic number stands for.
Magic numbers are constants that appear out of nowhere in your code and leave future readers (again, possibly future you) completely confused.
Example:
# Bad: What does 60 mean? if speed > 60: print("You're speeding!") # Good: Define constants SPEED_LIMIT = 60 if speed > SPEED_LIMIT: print("You're speeding!")
Pro tip: If you see a number in your code, slap a constant on it. Constants are like your GPS—no one likes being lost without them.
6. Handle Errors Gracefully 💥
No one likes a program that crashes as soon as something unexpected happens. A well-written program handles errors gracefully, gives useful feedback, and lives to fight another day.
Example:
# Bad: This will crash if order is None def process_order(order): total = order['total'] # Good: Handle the error gracefully def process_order(order): if order is None: raise ValueError("Order cannot be None") total = order['total']
Imagine your code is like a chef. When something goes wrong, it should calmly say, “No worries, we’ll fix this,” instead of throwing the whole kitchen into chaos.
7. Be Consistent with Formatting 🎨
If your codebase looks like it was formatted by a squirrel on caffeine, it’s going to be hard to maintain. Consistency in formatting (indentation, spacing, naming conventions) is key. Use tools like black or flake8 in Python to keep things neat and uniform.
# Run the black formatter black my_code.py
Your code should look as clean as a well-organized desk, not like a junk drawer with random bits all over the place.
8. Test Your Code ✅
If you’re not writing tests for your code, you’re basically flying a plane without checking if the wings are still attached. Unit tests, integration tests, and functional tests ensure your code does what it’s supposed to do.
Example:
import unittest class TestOrderProcessing(unittest.TestCase): def test_calculate_total(self): self.assertEqual(calculate_total([10, 20, 30]), 60) if __name__ == '__main__': unittest.main()
Write tests like your future paycheck depends on it—because it probably does. And don’t forget: test-driven development is your friend, even if it seems like extra work up front.
9. Refactor Often 🔄
You’ve written your code and it works. Great! But can it be better? Refactoring is the process of cleaning up and improving the structure of your code without changing its functionality. It’s like decluttering your code closet—get rid of the mess and keep only what’s essential.
Example:
# Before refactor def apply_discount(price, discount): if discount == 0: return price return price - (price * discount) # After refactor: cleaner, simpler def apply_discount(price, discount): return price if discount == 0 else price - (price * discount)
Regularly refactor your code, because no one likes a hoarder—not even in code.
10. Collaborate with Version Control 🧑🤝🧑
If you’re working on a team (or even solo), version control is a must. Tools like Git allow you to track changes, collaborate with others, and avoid the dreaded situation where someone overwrites your code.
Branch early, branch often, and remember to commit meaningful messages. None of this "fix stuff" nonsense!
Example:
git commit -m "chore(shop): Refactored discount logic to improve readability"
Your codebase should feel like a well-rehearsed orchestra, not a free-for-all jam session.
Conclusion: Clean Code for a Cleaner Mind 🧠✨
Writing clean, maintainable code is not just about making your code prettier—it’s about making your life (and your teammates' lives) easier in the long run. By following these 10 secrets, you’ll write code that’s easy to read, easy to debug, and, most importantly, easy to maintain.
Now, go forth and code like a tidy wizard 🧙♂️, leaving no trace of chaotic spells behind!
Did You Enjoy the Blog? 👏
If these tips helped you, give this blog a clap! Your support helps us continue sharing valuable tips.
Share it with your friends and network—spread the wisdom of clean code far and wide! Every share helps us grow and allows us to add more awesome content.
Thank you for reading and happy coding! 😄