A Beginner’s Journey Into FastAPI: The Fast Lane of Python Web Frameworks
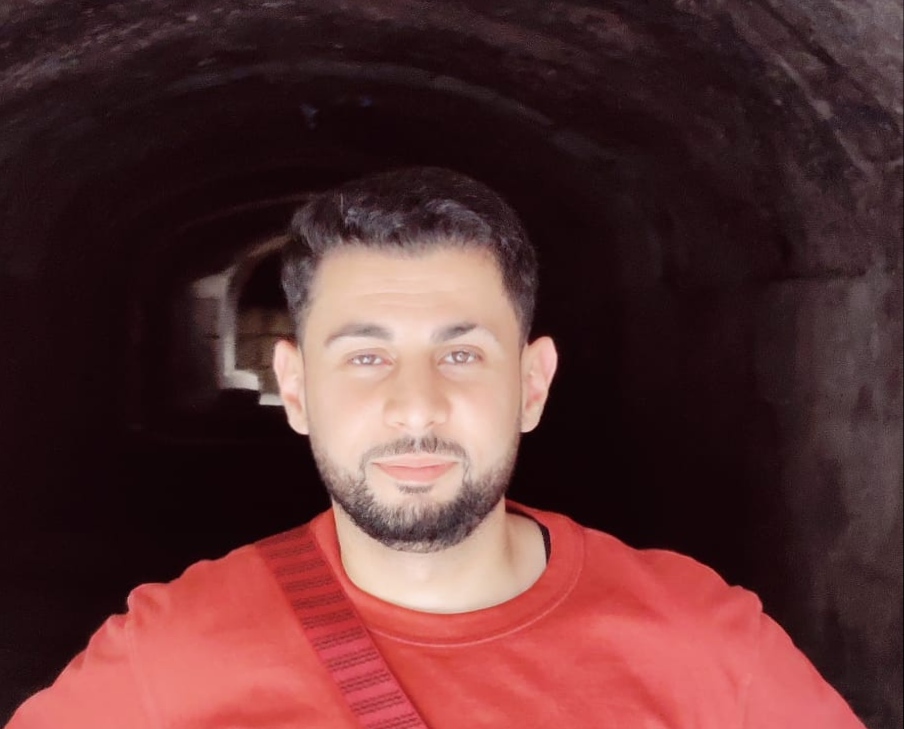
MontaF - Oct. 11, 2024
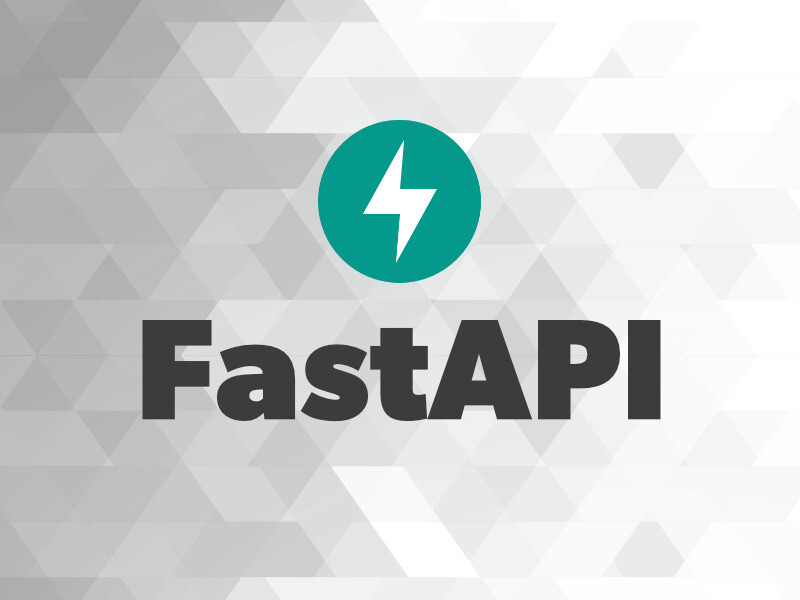
So, you’ve heard about this new shiny thing called FastAPI, and you’re wondering what all the fuss is about. Maybe you’ve dabbled in Flask or Django, and you’ve heard that FastAPI is the Usain Bolt of web frameworks—fast, modern, and cool. Well, buckle up because we’re about to take a ride down the highway of FastAPI development! Don’t worry, there will be jokes, speed, and code, but no speed traps or bugs (hopefully).
What Is FastAPI ?
FastAPI is a modern web framework for building APIs with Python 3.7+ that is fast, easy to use, and very developer-friendly. It comes with a host of features designed to make your life easier:
- Automatic interactive documentation (thanks to OpenAPI and Swagger)
- High performance (it’s powered by the fast-as-a-cheetah ASGI)
- Type hints (making Python type checking more than just a pretty suggestion)
- Data validation (because who needs surprises in their API payloads?)
FastAPI makes building APIs as smooth as butter on toast. It’s like Django’s hip, younger sibling who moved to the big city and now wears sunglasses indoors. And did I mention it’s FAST? (The "Fast" in FastAPI isn’t there for decoration).
Why FastAPI Is Awesome
FastAPI's standout features include:
- Blazing Speed 🚀: It’s as fast as Node.js and Go, thanks to asynchronous programming with Python's async/await.
- Type Hints 🧠: It uses Python's type hints to make code more readable, and it validates request data out of the box.
- Interactive Documentation 📄: Automatically generates documentation with Swagger UI and Redoc. So, you can look cool while showing off your API’s capabilities.
- Ease of Use 🤝: Get started fast (pun intended) without a steep learning curve.
- Starlette + Pydantic Power Combo 💪: It’s built on Starlette (for web handling) and Pydantic (for data validation). That’s like having two superheroes watch your back.
Now that we’ve covered why FastAPI rocks, let’s hit the accelerator and start coding!
Setting Up FastAPI
First, make sure you have Python 3.7+ installed. Then, create a virtual environment (because you don't want your project dependencies spilling all over your machine like glitter).
python3 -m venv venv
source venv/bin/activate
Next, install FastAPI and a server to run it on, like Uvicorn (the fastest mythical beast of web servers):
pip install fastapi uvicorn
And just like that, you’re ready to roll.
Your First FastAPI App
Let’s build your first API with FastAPI. Spoiler alert: It’s going to be ridiculously easy.
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def read_root():
return {"message": "Hello, FastAPI!"}
This tiny app has already created a GET endpoint for you at the root ("/"). If you squint, it almost looks too simple to be true, but it is.
Now, fire up Uvicorn to run your API:
uvicorn main:app --reload
Visit http://127.0.0.1:8000/
in your browser, and boom, there’s your API spitting out JSON like a pro!
Automatic Interactive Documentation
Remember when I mentioned interactive docs? Here’s where FastAPI flexes its Swagger-powered muscles. Head over to:
http://127.0.0.1:8000/docs
You’ll see a beautifully interactive Swagger UI. It’s so cool that you’ll be tempted to call your boss just to show it off (don’t, unless they’re paying you extra).
Or, if you like your docs with a little more sophistication (think API documentation in a suit and tie), check out:
http://127.0.0.1:8000/redoc
Redoc will present your API documentation in a more formal, minimalist style. You’ll feel like you’ve just entered an API art gallery.
A Quick API Example: CRUD with FastAPI
Time to build something slightly more useful—let’s do a simple CRUD API for a book collection. Every developer’s rite of passage!
from typing import List
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class Book(BaseModel):
title: str
author: str
pages: int
books = []
@app.post("/books/", response_model=Book)
def create_book(book: Book):
books.append(book)
return book
@app.get("/books/", response_model=List[Book])
def read_books():
return books
@app.get("/books/{book_id}")
def read_book(book_id: int):
return books[book_id]
@app.delete("/books/{book_id}")
def delete_book(book_id: int):
books.pop(book_id)
return {"message": "Book deleted"}
Breaking Down the Code:
Book
: A Pydantic model that defines how our book data should look. Thanks to Pydantic, if someone tries to submit a book without an author, we won’t have to deal with any drama.POST /books/
: Allows you to add a new book to the collection.GET /books/
: Fetches a list of all the books (because knowledge is power).GET /books/{book_id}
: Retrieves a specific book by ID.DELETE /books/{book_id}
: Deletes a book (RIP).
And just like that, you've built a fully functioning API with four endpoints—in less than 20 lines of code. That’s some fast coding! 🚗💨
Asynchronous Goodness
FastAPI also supports asynchronous code out of the box. Let’s say you want to make your book API more efficient when fetching large amounts of data. Just slap async
onto your function, and you’re off to the races!
@app.get("/books/", response_model=List[Book])
async def read_books():
return books
What’s Happening Under the Hood?
FastAPI leverages Python's type hints to automatically validate and serialize data. If you try to submit invalid data, FastAPI will tell you what’s wrong before your server even has a chance to freak out.
Behind the scenes, it uses the power of Starlette for asynchronous request handling and Pydantic for data validation, which makes it lightning-fast and developer-friendly. It also uses JSON Schema for generating OpenAPI docs, so your API is always documented.
Error Handling Like a Pro
You didn’t think we’d go the whole Blog without mentioning error handling, did you? FastAPI makes dealing with errors super simple.
from fastapi import HTTPException
@app.get("/books/{book_id}")
def read_book(book_id: int):
if book_id >= len(books):
raise HTTPException(status_code=404, detail="Book not found")
return books[book_id]
With this, your API will return a nice 404 response when a book isn’t found. Your users will appreciate you not leaving them hanging with a cryptic error message.
Conclusion: Why FastAPI Is the Future (And You Should Learn It Yesterday)
If you’re serious about building high-performance, modern web applications, FastAPI is the way to go. It's fast, async-ready, and ridiculously easy to use. Plus, the built-in interactive docs make you look like an API wizard when demoing to clients or teammates.
So, why not join the FastAPI revolution? You’ll spend less time coding and more time sipping coffee while Swagger does the hard work of showing off your APIs. 🚀
Now, go forth and build the fastest, most well-documented APIs your users have ever seen!
Happy coding 😊