Implementing Role-Based Access Control (RBAC) for APIs
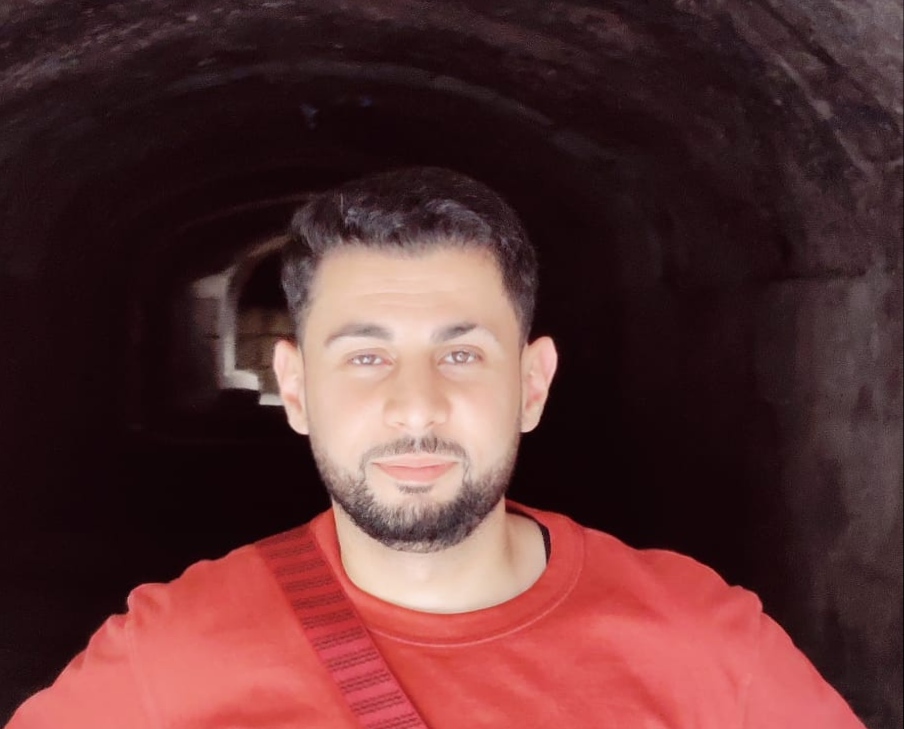
MontaF - Nov. 17, 2024
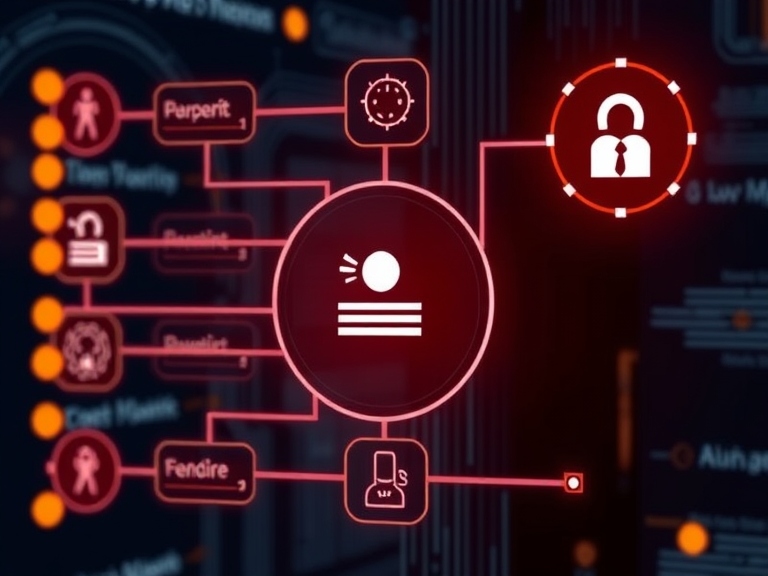
APIs are the backbone of modern applications, serving as the bridge between frontends, services, and data. As APIs scale and deal with sensitive operations, securing them becomes crucial. One effective strategy is Role-Based Access Control (RBAC). In this post, we’ll explore what RBAC is, why it’s vital, and how to implement it for APIs in a practical way—with code snippets along the journey.
What is RBAC?
RBAC is a security model that restricts system access based on roles assigned to users. In simpler terms:
- Roles: Groups of permissions.
- Users: Individuals or entities that are assigned roles.
- Permissions: Actions or resources users can access.
For example:
- A reader role might only allow viewing data.
- An admin role might allow creating, editing, and deleting data.
Here’s a conceptual diagram:
Why Use RBAC for APIs?
Implementing RBAC has several benefits for API security:
- Granular Access Control: Define who can do what, reducing misuse or accidental data breaches.
- Ease of Maintenance: Centralized roles and permissions make managing user access simpler.
- Scalability: As the number of API endpoints grows, RBAC scales easily by updating roles instead of individual permissions.
Core Concepts for Implementing RBAC
To implement RBAC in APIs, you’ll need:
- User Authentication: Verify the user’s identity (e.g., using OAuth or JSON Web Tokens - JWT).
- Role Assignment: Assign specific roles to users.
- Permission Mapping: Map roles to actions and resources.
- Access Enforcement: Validate user roles before allowing access to API endpoints.
Step-by-Step Guide to RBAC Implementation
Let’s build a simple RBAC system using Node.js and Express.js. For this, we’ll assume you have basic knowledge of JavaScript and REST APIs.
1. Set Up Your Project
First, create a new Node.js project:
mkdir rbac-api cd rbac-api npm init -y npm install express jsonwebtoken dotenv
Set up a basic Express server in server.js
:
const express = require('express'); const app = express(); app.use(express.json()); app.get('/', (req, res) => res.send('RBAC API is running!')); const PORT = process.env.PORT || 3000; app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
2. Define Roles and Permissions
Create a roles.js
file to define your roles and their permissions:
const roles = { admin: ['create', 'read', 'update', 'delete'], editor: ['read', 'update'], viewer: ['read'] }; module.exports = roles;
3. Simulate User Data
For simplicity, create a users.js
file with mock user data:
const users = [ { id: 1, username: 'alice', role: 'admin' }, { id: 2, username: 'bob', role: 'editor' }, { id: 3, username: 'carol', role: 'viewer' } ]; module.exports = users;
4. Add Middleware for Authentication
In server.js
, add authentication logic using JWT. Install jsonwebtoken
if you haven’t already:
npm install jsonwebtoken
Update server.js
:
const jwt = require('jsonwebtoken'); const roles = require('./roles'); const users = require('./users'); const SECRET_KEY = 'your-secret-key'; // Middleware to authenticate users const authenticate = (req, res, next) => { const token = req.header('Authorization'); if (!token) return res.status(401).send('Access denied. No token provided.'); try { const decoded = jwt.verify(token, SECRET_KEY); req.user = decoded; next(); } catch (err) { res.status(400).send('Invalid token.'); } };
5. Add Role Validation
Next, enforce role-based access with middleware:
// Middleware to authorize based on roles const authorize = (requiredRole) => { return (req, res, next) => { const userRole = req.user.role; if (!roles[userRole].includes(requiredRole)) { return res.status(403).send('Access forbidden: insufficient permissions.'); } next(); }; };
6. Secure Your Endpoints
Secure endpoints by combining authentication and authorization middleware:
// Protected route example app.post('/data', authenticate, authorize('create'), (req, res) => { res.send('Data created successfully!'); }); app.get('/data', authenticate, authorize('read'), (req, res) => { res.send('Here is the data!'); });
7. Generate JWT Tokens for Users
Simulate login and JWT generation for users:
app.post('/login', (req, res) => { const { username } = req.body; const user = users.find(u => u.username === username); if (!user) return res.status(400).send('Invalid username.'); const token = jwt.sign({ id: user.id, role: user.role }, SECRET_KEY); res.send({ token }); });
Testing the Implementation
Start the server:
node server.js
Simulate a login to get a JWT token:
curl -X POST -H "Content-Type: application/json" -d '{"username": "alice"}' http://localhost:3000/login
This returns a token:
{ "token": "your.jwt.token.here" }
Use the token to access protected routes:
curl -X POST -H "Authorization: your.jwt.token.here" http://localhost:3000/data
Enhancements and Best Practices
- Dynamic Role Management: Store roles in a database to allow dynamic updates.
- Audit Logs: Log access attempts for security audits.
- Testing: Use tools like Postman or automated tests to validate role permissions.
- Scalability: Use frameworks like Keycloak or AWS IAM for enterprise-grade RBAC.
Conclusion
Implementing Role-Based Access Control (RBAC) for APIs is critical for maintaining security and scalability. By systematically defining roles, permissions, and access enforcement, you can secure your APIs effectively.
With the practical steps and code examples above, you should have a solid foundation to start implementing RBAC in your projects.
Happy coding!