Getting Started with JavaScript: A Beginner’s Guide
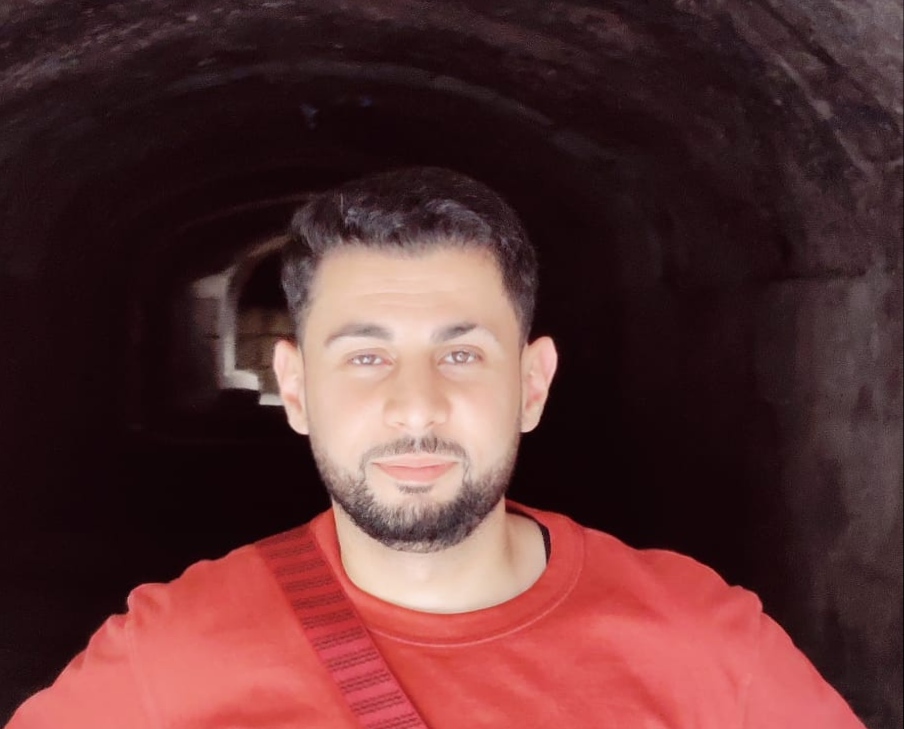
MontaF - Sept. 1, 2024
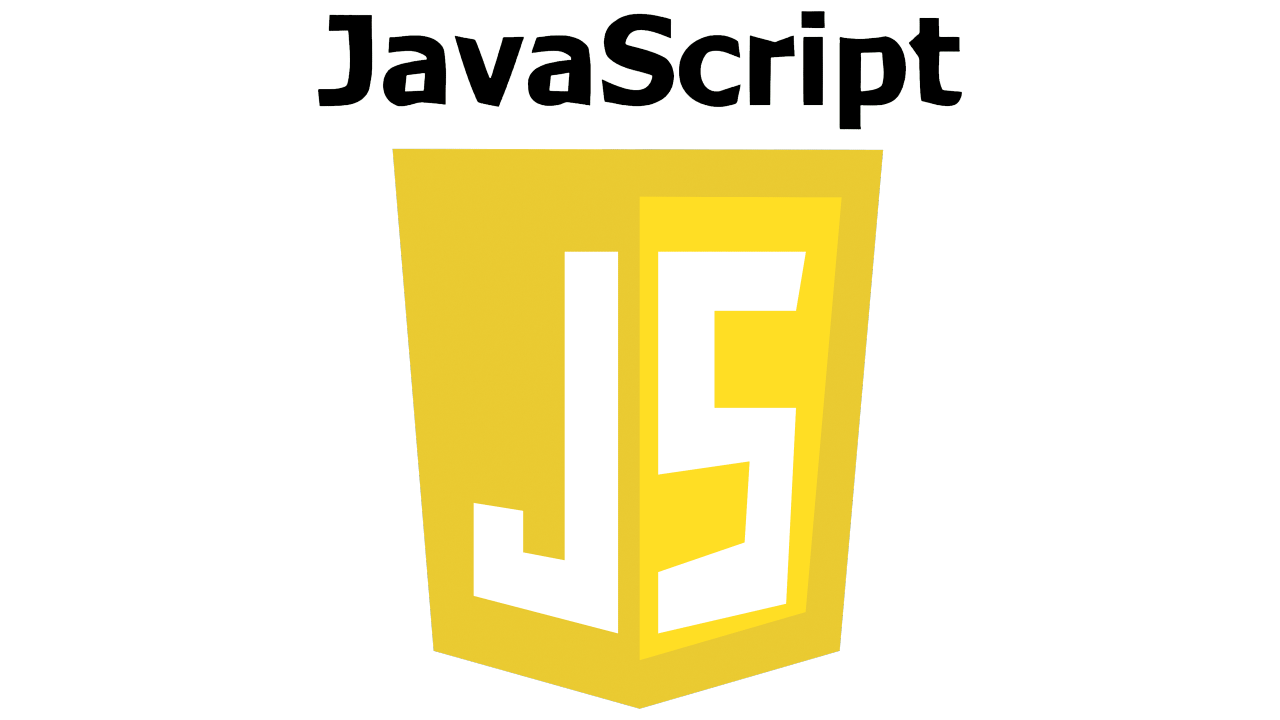
JavaScript is one of the most widely used programming languages in the world, particularly in web development.
It allows you to create interactive and dynamic web pages, and with the rise of frameworks like React, Angular, and Node.js, JavaScript has become a full-stack language capable of powering entire web applications.
In this guide, we'll introduce you to the basics of JavaScript, show you how to set up your environment, and walk you through writing your first JavaScript code.
Why Learn JavaScript?
JavaScript is an essential language for anyone interested in web development. Here’s why you should consider learning it:
- Front-End Development: JavaScript is the language of the web, allowing you to create interactive and responsive user interfaces.
- Back-End Development: With environments like Node.js, you can use JavaScript on the server side as well, making it possible to use a single language across the entire stack.
- Vast Ecosystem: JavaScript has a massive ecosystem of libraries, frameworks, and tools that make development faster and easier.
- High Demand: JavaScript is consistently one of the most in-demand programming languages in the job market.
Step 1: Setting Up Your Environment
Before you can start coding in JavaScript, you need to set up a development environment. Fortunately, getting started with JavaScript is easy, as it runs directly in your web browser.
Choosing a Text Editor
To write JavaScript code, you’ll need a text editor. Here are some popular choices:
- Visual Studio Code: A free, powerful, and widely-used editor with built-in support for JavaScript.
- Sublime Text: A lightweight editor with a simple interface.
- Atom: A free and open-source editor developed by GitHub.
Using the Browser Console
Every modern web browser comes with a built-in JavaScript console, which is great for testing out small pieces of code.
- Open the Console:
- In Google Chrome, press
Ctrl + Shift + J
(Windows/Linux) orCmd + Option + J
(macOS). - In Firefox, press
Ctrl + Shift + K
(Windows/Linux) orCmd + Option + K
(macOS).
- Run JavaScript Code:
- You can type JavaScript code directly into the console and see the results immediately.
Step 2: Writing Your First JavaScript Program
Let’s start with the classic “Hello, World!” example, a simple program that displays a message on the screen.
Using HTML and JavaScript Together
JavaScript is often embedded in HTML to create dynamic web pages. Here’s how to write a basic HTML file with embedded JavaScript.
1.Create an HTML File:
Open your text editor and create a new file named index.html
.
Add the following code:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Hello, World!</title> </head> <body> <h1>Hello, World!</h1> <script> console.log("Hello, World from JavaScript!"); </script> </body> </html>
2.Open the HTML File:
- Save the file and open it in your web browser. You should see “Hello, World!” displayed on the page.
- Open the browser console (as described earlier) to see the message “Hello, World from JavaScript!” logged by the JavaScript code.
Using External JavaScript Files
You can also write JavaScript in a separate file and link it to your HTML file.
1.Create a JavaScript File:
In your text editor, create a new file named script.js
.
Add the following code::
console.log("Hello, World from external JavaScript!");
2.Modify the HTML File:
Update your index.html
file to include the external JavaScript file:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Hello, World!</title> </head> <body> <h1>Hello, World!</h1> <script src="script.js"></script> </body> </html>
3.View the Output:
Save both files and open index.html
in your browser. Check the console to see the “Hello, World from external JavaScript!” message.
Step 3: Understanding JavaScript Basics
Now that you’ve written your first JavaScript program, let’s dive into some fundamental concepts.
Variables and Data Types:
Variables in JavaScript are used to store data. You can declare variables using let
, const
, or var
.
let name = "Alice"; // String const age = 30; // Number let isStudent = true; // Boolean console.log(name, age, isStudent);
Operators:
JavaScript supports various operators for performing operations like addition, subtraction, and comparison.
let a = 10; let b = 5; console.log(a + b); // Addition console.log(a - b); // Subtraction console.log(a * b); // Multiplication console.log(a / b); // Division console.log(a > b); // Greater than
Functions:
Functions allow you to encapsulate code into reusable blocks.
function greet(name) { return "Hello, " + name; } let message = greet("Alice"); console.log(message);
Arrays:
Arrays are used to store multiple values in a single variable.
let fruits = ["Apple", "Banana", "Cherry"]; console.log(fruits[0]); // Accessing the first element console.log(fruits.length); // Getting the length of the array
Objects:
Objects are collections of related data and functionality, represented as key-value pairs.
let person = { name: "Alice", age: 30, greet: function() { return "Hello, " + this.name; } }; console.log(person.name); // Accessing properties console.log(person.greet()); // Calling methods
Step 4: Manipulating the DOM
The Document Object Model (DOM) is a representation of the HTML structure of a webpage.
JavaScript allows you to interact with and manipulate the DOM to change the content and appearance of a webpage dynamically.
Selecting Elements:
You can select HTML elements using methods like getElementById
or querySelector
.
// Selecting an element by ID let heading = document.getElementById("main-heading"); console.log(heading.textContent); // Selecting an element by query selector let paragraph = document.querySelector(".intro"); console.log(paragraph.textContent);
Changing Content:
You can change the content of an HTML element using properties like textContent
or innerHTML
.
let heading = document.getElementById("main-heading"); heading.textContent = "Welcome to JavaScript!";
Adding Event Listeners:
JavaScript allows you to make your web pages interactive by responding to user actions, like clicks or key presses.
let button = document.getElementById("myButton"); button.addEventListener("click", function() { alert("Button was clicked!"); });
Step 5: Debugging JavaScript Code
Debugging is an essential skill in programming. Here are some tips for debugging JavaScript:
Using console.log
:
One of the simplest ways to debug your code is by printing values to the console using console.log
.
let x = 10; console.log("The value of x is:", x);
Using Browser Developer Tools:
Modern browsers come with developer tools that provide powerful debugging features:
- Breakpoints: You can set breakpoints in your code, allowing you to pause execution and inspect variables.
- Watch Expressions: Monitor the values of specific variables as your code runs.
- Call Stack: See the order in which functions are called.
To access these tools, open your browser’s developer tools (usually by pressing F12
) and navigate to the “Sources” tab.
Step 6: Next Steps
You’ve just scratched the surface of what JavaScript can do. Here are some next steps to continue your learning:
- Learn More About ES6 Features: Modern JavaScript (ES6 and later) introduces new features like arrow functions, template literals, destructuring, and more.
- Explore JavaScript Libraries and Frameworks: Popular libraries and frameworks like React, Angular, and Vue.js can help you build more complex and efficient web applications.
- Practice by Building Projects: Start small projects like a to-do list app, a simple game, or a weather dashboard to practice your skills.
- Dive Into Asynchronous JavaScript: Learn about promises, async/await, and how to handle asynchronous operations like fetching data from an API.
Conclusion
JavaScript is a powerful and versatile language that plays a crucial role in modern web development.
By mastering the basics, you’ve taken the first step toward becoming a proficient JavaScript developer.
Keep practicing, explore.